How to Create a NuGet Package with References to Other Projects in the Same Solution
Introduction
Hello, devs! In the world of software development, efficiently sharing code is essential for productivity and project maintenance. One of the most popular methods for this is through NuGet packages.
In this post, we will explore how to create a NuGet package that includes references to other projects within the same solution, ensuring you can distribute your libraries effectively and organized.
Let’s go?
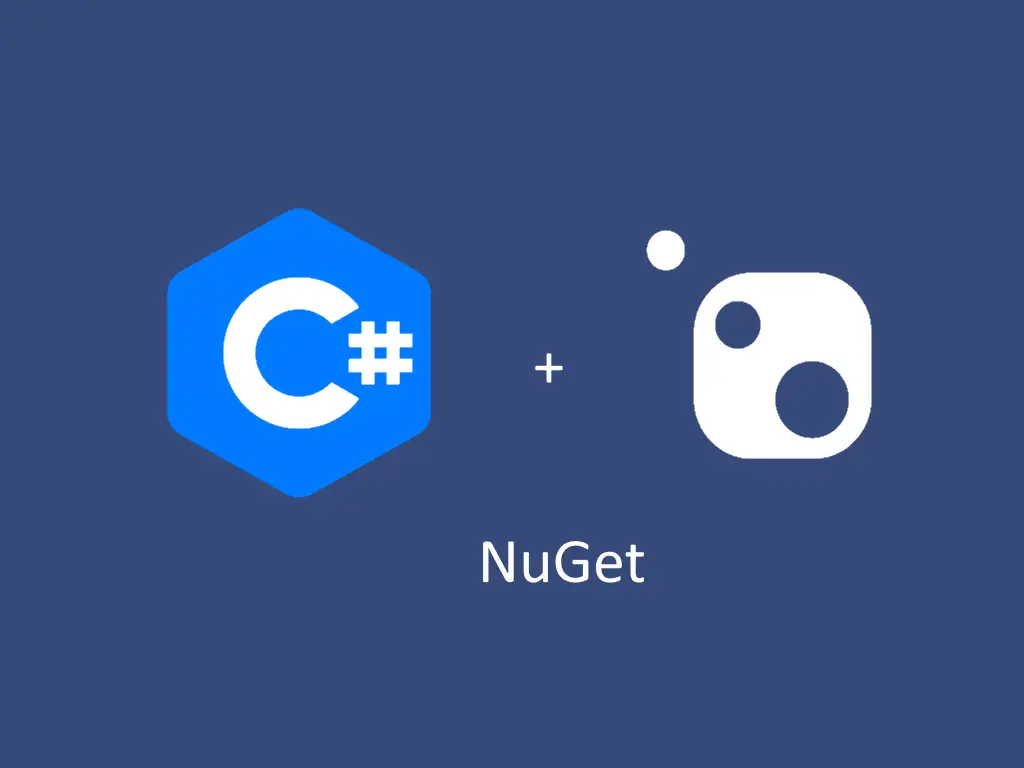
Understanding the Scenario
Imagine working on a solution that contains multiple projects. One of these projects directly depends on another within the same solution.
To simplify management and distribution, you decide to create a NuGet package.
However, when packaging your project, you realize you need to include the correct references to ensure everything works when the package is used in another solution.
How can we do this?
Step-by-Step Guide to Creating the NuGet Package
Project Configuration
First, ensure all projects in your solution are correctly configured. Each project should have a well-defined .csproj file with all dependencies and references added.
Modifications to the .csproj File
To include references to other projects in the same solution and ensure the NuGet package is generated correctly, make some modifications to the main project’s .csproj file.
Add the following snippet within the <PropertyGroup> element:
<PropertyGroup>
<TargetsForTfmSpecificBuildOutput>$(TargetsForTfmSpecificBuildOutput);CopyProjectReferencesToPackage</TargetsForTfmSpecificBuildOutput>
</PropertyGroup>
Next, add the reference to the dependent project with the necessary properties to include the output assembly:
<ItemGroup>
<ProjectReference Include="..\DependencyProject\DependencyProject.csproj">
<ReferenceOutputAssembly>true</ReferenceOutputAssembly>
<IncludeAssets>DependencyProject.dll</IncludeAssets>
</ProjectReference>
</ItemGroup>
Finally, include the following target within the <Project> tags:
<Target DependsOnTargets="ResolveReferences" Name="CopyProjectReferencesToPackage">
<ItemGroup>
<BuildOutputInPackage Include="@(ReferenceCopyLocalPaths->WithMetadataValue('ReferenceSourceTarget', 'ProjectReference'))"/>
</ItemGroup>
</Target>
Complete .csproj File Example
Here is a complete example of a .csproj file configured to include references to other projects and ready for packaging:
<Project Sdk="Microsoft.NET.Sdk">
<PropertyGroup>
<TargetFramework>net8.0</TargetFramework>
<Version>1.0.0</Version>
<Product>MyProduct</Product>
<PackageId>MyProduct</PackageId>
<Authors>YourName</Authors>
<Company>CompanyName</Company>
<Description>Package Description</Description>
<Copyright>Copyright © 2024 MyCompany</Copyright>
<TargetsForTfmSpecificBuildOutput>$(TargetsForTfmSpecificBuildOutput);CopyProjectReferencesToPackage</TargetsForTfmSpecificBuildOutput>
</PropertyGroup>
<ItemGroup>
<PackageReference Include="SomeNugetPackage" Version="1.2.3"/>
</ItemGroup>
<ItemGroup>
<ProjectReference Include="..\DependencyProject\DependencyProject.csproj">
<ReferenceOutputAssembly>true</ReferenceOutputAssembly>
<IncludeAssets>DependencyProject.dll</IncludeAssets>
</ProjectReference>
</ItemGroup>
<Target DependsOnTargets="ResolveReferences" Name="CopyProjectReferencesToPackage">
<ItemGroup>
<BuildOutputInPackage Include="@(ReferenceCopyLocalPaths->WithMetadataValue('ReferenceSourceTarget', 'ProjectReference'))"/>
</ItemGroup>
</Target>
</Project>
Adding an External DLL
Now that we have learned to add DLLs that are in the same solution, how would we add just an external DLL that is not a project?
You can use the following snippet in your .csproj file:
<ItemGroup>
<Content Include="<path to other dll>">
<Pack>true</Pack>
<PackagePath>lib\$(TargetFramework)</PackagePath>
</Content>
</ItemGroup>
This code ensures that the external DLL will be included in the NuGet package, making it available to any project consuming the package.
Conclusion and Final Tips
Creating a NuGet package with references to other projects in the same solution may seem complicated at first glance, but with the correct configuration, the process becomes much simpler. Always ensure that all dependencies are properly included and tested.
I hope this guide has been helpful and that you are ready to create your own NuGet packages with multiple dependencies.
See ya!