Dealing with Concurrency in Redis in Distributed Environments with C# and .NET
Introduction
Hello, devs!
Have you ever wondered how to handle concurrency when using Redis in a distributed environment? If so, you’re in the right place.
Today, we’ll explore the importance of Redis, its utilities, and, most importantly, how to solve concurrency problems with C# and .NET. We’ll use a practical code example to make everything clear.
Let’s go?
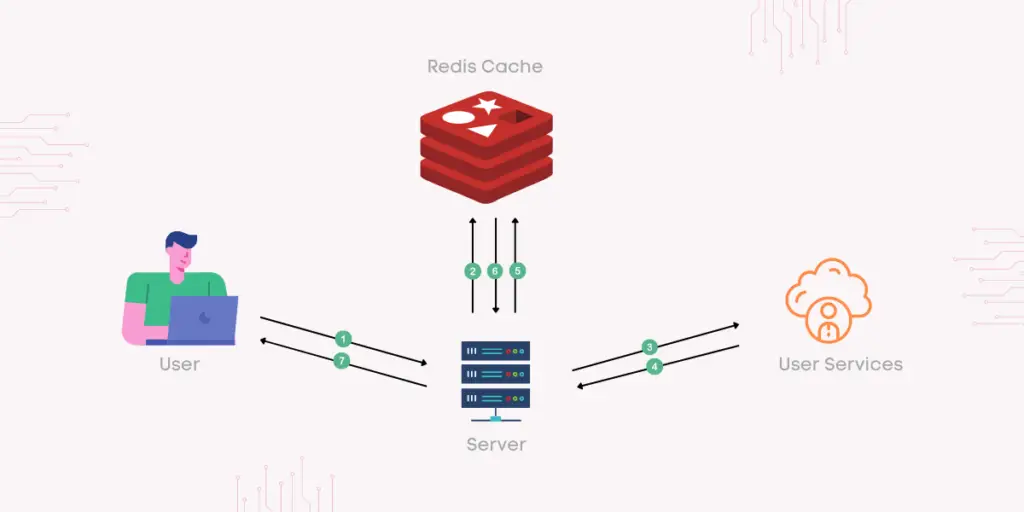
What is Redis and Its Importance
Redis is an in-memory database, extremely fast, used for caching, session management, queues, and much more.
Its popularity is due to its performance and versatility, supporting various data structures like strings, hashes, lists, sets, and sorted sets.
Using Redis can significantly improve your application’s performance by reducing the load on the main database, speeding up access to frequently used data.
Concurrency Challenges in Distributed Environments
In distributed environments, concurrency is a common challenge.
When multiple instances of an application try to access and modify the same data simultaneously, issues like race conditions can occur.
These issues can lead to data inconsistencies, compromising the integrity and reliability of the application.
Handling concurrency involves ensuring that only one instance of the application can modify a specific piece of data at a time, using locking mechanisms.
Using Redis to Handle Concurrency
Redis offers locking commands, such as SETNX
and GETSET
, but the LOCK command is particularly useful for avoiding race conditions.
Using LOCK
, we can ensure that only one instance of the application can execute a critical operation at a time.
Additionally, implementing exponential backoff for retries can help reduce contention and improve application performance.
Practical Example with C# and .NET
Let’s examine a C# code example that demonstrates how to handle concurrency using Redis in a distributed environment.
The code below attempts to add a processed message to the cache using a locking mechanism to ensure that only one instance can do so at a time:
public async Task<bool> TryToAddProcessedMessageToCache(int messageId, int count = 0)
{
const int maxRetries = 10;
const int baseDelayMilliseconds = 50;
var lockKey = $"lock:{messageId}";
var lockExpiration = TimeSpan.FromSeconds(3);
var token = Guid.NewGuid().ToString();
bool lockSucceeded = false;
try
{
lockSucceeded = await _redisDatabase.LockTakeAsync(lockKey, token, lockExpiration);
if (lockSucceeded)
{
var messageKey = $"message:{messageId}";
var messageExpiration = TimeSpan.FromSeconds(30);
var hasToProcessMessage = await _redisDatabase.StringSetAsync(messageKey, "processed", when: When.NotExists, expiry: messageExpiration);
return hasToProcessMessage;
}
}
catch (Exception)
{
throw;
}
finally
{
if (lockSucceeded)
{
await _redisDatabase.LockReleaseAsync(lockKey, token);
}
}
// If the lock was already active or an error occurred
if (count < maxRetries)
{
count++;
var sleepTime = TimeSpan.FromMilliseconds(count * baseDelayMilliseconds);
await Task.Delay(sleepTime);
return await TryToAddProcessedMessageToCache(messageId, count);
}
return false;
}
In the code above, we use LockTakeAsync
to try to acquire an exclusive lock for the message identified by messageId
.
If the lock is acquired, the message is marked as processed in the cache.
After the operation, the lock is released with LockReleaseAsync
.
If the lock is not acquired, the method retries with increasing delay, until it reaches the maximum number of attempts.
Best Practices and Additional Tips
- Avoid Long Locks: Keep the lock duration as short as possible to reduce contention;
- Exponential Backoff: Use exponential backoff for retries, as shown in the example, to avoid overloading the system;
- Monitoring: Implement monitoring and logging to quickly identify and resolve concurrency issues;
- Extensive Testing: Conduct load testing to ensure that your locking mechanism works correctly under different concurrency conditions.
Conclusion
Handling concurrency in distributed environments can be challenging, but with the right tools and techniques, such as Redis and locks, you can ensure data integrity and application performance.
Try implementing the provided code example and adjust it according to your project’s needs.
If you have any questions or suggestions, leave a comment below!
See ya!