Best Practices in REST API: Exploring HTTP Verbs with C# Examples
Introduction
Hello, devs! REST APIs are the backbone of modern web communication.
In this post, we will explore best practices for creating RESTful APIs and understand the differences between HTTP verbs with practical examples in C#.
We’ll use an order and item structure as examples to make everything more practical and applicable to your daily development.
Let’s go?
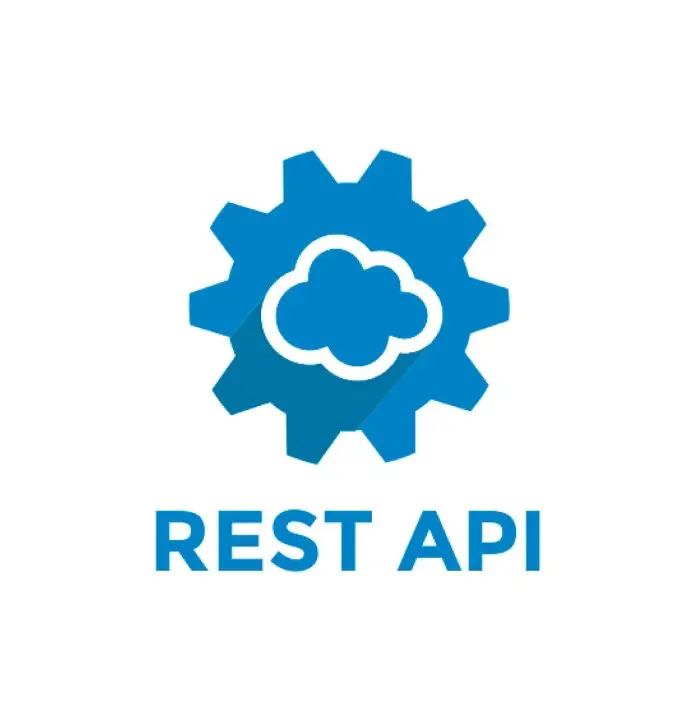
What is a REST API?
A REST API (Representational State Transfer) is a set of rules that allows communication between systems using the HTTP protocol.
They are widely used due to their simplicity and ability to integrate different systems efficiently.
Key characteristics of a RESTful API:
- Stateless: Each API call contains all the information necessary for the server to understand and process the request;
- Uniform Interface: Defines a consistent contract for the API, typically using predictable endpoints and standard HTTP methods;
- Client-Server Architecture: The architecture divides the client (user interface) and the server (backend and database) to promote scalability;
Best Practices for REST APIs
Endpoint Design:
-
Use nouns for endpoints and verbs for HTTP methods. For example,
/orders
to access orders; -
Follow a consistent URL pattern. For example,
/orders/{orderId}/items
to access items of a specific order;
API Versioning:
-
Include the API version in the URL, such as
/api/v1/orders
, to facilitate maintenance and updates;
Authentication and Authorization:
- Use JWT tokens for authentication;
- Implement access control to ensure users can only access resources they are permitted to;
Error Handling:
- Use appropriate HTTP status codes;
- Provide clear and detailed error messages;
HTTP Verbs and Their Uses
GET: Retrieving data
- Used to retrieve information about a resource;
-
Example:
GET /orders
to list all orders.
POST: Creating new resources
- Used to create a new resource;
-
Example:
POST /orders
to create a new order.
PUT: Updating existing resources
- Used to replace an existing resource;
-
Example:
PUT /orders/{id}
to update an entire order.
PATCH: Partial update of resources
- Used to partially update a resource;
-
Example:
PATCH /orders/{orderId}/items/{itemId}
to update a specific field of an order item.
DELETE: Removing resources
- Used to remove a resource;
-
Example:
DELETE /orders/{orderId}/items/{itemId}
to delete an item from an order
Code Examples in C#
Let’s examine a C# code example that demonstrates how to use a REST API.
- Orders:
[ApiController]
[Route("api/v1/orders")]
public class OrdersController : ControllerBase
{
[HttpGet]
public async Task<IActionResult> GetOrders()
{
// Call logic to retrieve orders
return Ok(/* list of orders */);
}
[HttpPost]
public async Task<IActionResult> CreateOrder([FromBody] Order order)
{
// Call logic to create order
return CreatedAtAction(nameof(GetOrderById), new { id = order.Id }, order);
}
[HttpGet("{id}")]
public async Task<IActionResult> GetOrderById(int id)
{
// Call logic to retrieve order by ID
return Ok(/* order */);
}
[HttpPut("{id}")]
public async Task<IActionResult> UpdateOrder(int id, [FromBody] Order updatedOrder)
{
if (id != updatedOrder.Id)
{
return BadRequest();
}
// Call logic to update order
return NoContent();
}
}
- Items:
[ApiController]
[Route("api/v1/orders/{orderId}/items")]
public class ItemsController : ControllerBase
{
[HttpGet]
public async Task<IActionResult> GetItems(int orderId)
{
// Call logic to retrieve order items
return Ok(/* list of items */);
}
[HttpPatch("{itemId}")]
public async Task<IActionResult> UpdateItemPartial(int orderId, int itemId, [FromBody] JsonPatchDocument<Item> patchDoc)
{
if (patchDoc == null)
{
return BadRequest();
}
// Call logic to partially update item
return NoContent();
}
[HttpDelete("{itemId}")]
public async Task<IActionResult> DeleteItem(int orderId, int itemId)
{
// Call logic to delete item
return NoContent();
}
}
Conclusion
Following best practices for developing RESTful APIs is essential to create robust and efficient systems.
Understanding and correctly using HTTP verbs, along with a good code structure, will ensure your API is easy to maintain and scale.
We hope these C# examples help you implement your own APIs effectively.
If you have any questions or suggestions, leave a comment below!
See ya!