Migrating to Success: Pros, Cons, and Best Practices for Migrations in .NET with C#
Introduction
Hello, devs!
Today, we’re going to talk about a crucial topic in .NET and C# development: migrations.
We’ll explore the pros and cons, essential commands, code examples, and, of course, best practices to help you master migrations in your project.
Let’s go?
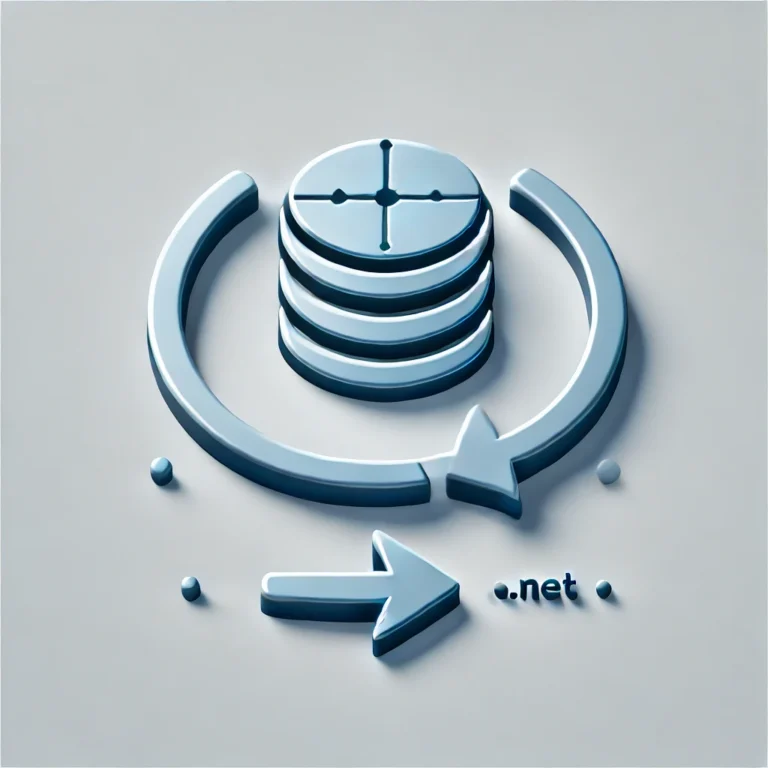
What are Migrations?
In a simple way, migrations are a powerful tool within Entity Framework that allows you to create, modify, and manage the database schema through code.
With migrations, you can track database changes throughout development, making it easier to maintain and evolve your project.
They are especially useful in agile development environments where frequent changes to the data schema are common.
Initial Setup
Adding Required Packages
To start using migrations in a .NET project, you need to add a few NuGet packages to your project. These packages include Entity Framework Core and design tools.
Run the following commands in the Package Manager Console to add the necessary packages:
Install-Package Microsoft.EntityFrameworkCore
Install-Package Microsoft.EntityFrameworkCore.Design
Install-Package Microsoft.EntityFrameworkCore.SqlServer
These packages include:
- Microsoft.EntityFrameworkCore: The main Entity Framework Core package.
- Microsoft.EntityFrameworkCore.Design: Design tools required to create and apply migrations.
- Microsoft.EntityFrameworkCore.SqlServer: Entity Framework Core provider for SQL Server.
Installing EF Core Tools
To use the dotnet ef
commands, you need to install the Entity Framework Core tools globally or as part of your project.
To install globally, run:
dotnet tool install --global dotnet-ef
Pros and Cons of Migrations
Advantages
- Ease of management: With migrations, you can apply and revert database changes in a controlled and replicable manner.
- Change history: Migrations maintain a detailed history of all changes made to the database, enabling efficient version control.
- Automation: You can automate the creation and application of migrations, integrating them into the build and deploy process.
Disadvantages
- Complexity in large environments: In large projects with many developers, managing migrations can become complex, requiring coordination and best practices to avoid conflicts.
- Conflict risks: Multiple developers working in different branches can create migration conflicts, necessitating good version and merge management.
Essential Migration Commands in .NET
Here are some of the most commonly used commands for working with migrations in .NET:
- Add Migration: Creates a new migration based on detected model changes.
dotnet ef migrations add InitialCreate
- Update Database: Applies pending migrations to the database.
dotnet ef database update
- Remove Migration: Removes the last created migration, if it has not been applied to the database.
dotnet ef migrations remove
- Script Migration: Generates an SQL script for the specified migration.
dotnet ef migrations script
Code Examples
Let’s see a practical example of how to create and apply migrations in a .NET project.
- Configuring the Context:
First, configure the Entity Framework context in your project:
public class ApplicationDbContext : DbContext
{
public DbSet<Customer> Customers { get; set; }
protected override void OnConfiguring(DbContextOptionsBuilder optionsBuilder)
{
optionsBuilder.UseSqlServer("YourConnectionStringHere");
}
}
- Defining the Customer Class:
Let’s define a simple Customer class with some properties and data annotations to configure the primary key, field sizes, and data types.
public class Customer
{
[Key]
[DatabaseGenerated(DatabaseGeneratedOption.Identity)]
public int Id { get; set; }
[Required]
[MaxLength(100)]
public string Name { get; set; }
public int Age { get; set; }
[Column(TypeName = "decimal(18,2)")]
public decimal Balance { get; set; }
[Required]
[EmailAddress]
public string Email { get; set; }
}
- Creating a Migration:
Create a new migration to add the Customers table:
dotnet ef migrations add AddCustomerTable
- Reviewing the Generated Migration:
The generated migration should look something like this:
public partial class AddCustomerTable : Migration
{
protected override void Up(MigrationBuilder migrationBuilder)
{
migrationBuilder.CreateTable(
name: "Customers",
columns: table => new
{
Id = table.Column<int>(nullable: false)
.Annotation("SqlServer:Identity", "1, 1"),
Name = table.Column<string>(maxLength: 100, nullable: false),
Age = table.Column<int>(nullable: false),
Balance = table.Column<decimal>(type: "decimal(18,2)", nullable: false),
Email = table.Column<string>(nullable: true)
},
constraints: table =>
{
table.PrimaryKey("PK_Customers", x => x.Id);
});
}
protected override void Down(MigrationBuilder migrationBuilder)
{
migrationBuilder.DropTable(
name: "Customers");
}
}
- Applying the Migration:
Apply the migration to update the database schema:
dotnet ef database update
Best Practices for Migrations
To ensure your migrations are managed efficiently, here are some best practices:
- Plan your migrations: Before creating a migration, plan the changes that will be made to the database. This helps avoid unnecessary migrations and conflicts.
- Use descriptive names: Name your migrations clearly and descriptively to make it easier to identify and understand the changes.
- Review migrations: Always review the code generated by migrations to ensure the changes are correct and will not negatively impact the database.
- Keep the database synchronized: Ensure that the local and production databases are synchronized to avoid issues during deployment.
- Integrate migrations into CI/CD: Automate the application of migrations in the CI/CD pipeline to ensure that database changes are applied consistently across all environments.
Conclusion
Migrations are a powerful tool in any .NET developer’s arsenal, allowing for efficient and controlled management of the database schema.
By following best practices and using essential commands, you can optimize the use of migrations in your project.
So don’t wait, start applying these tips today!
Now, you know a lit bit more about Migrations, let me know your thoughts about it. Did you like it?
See ya!